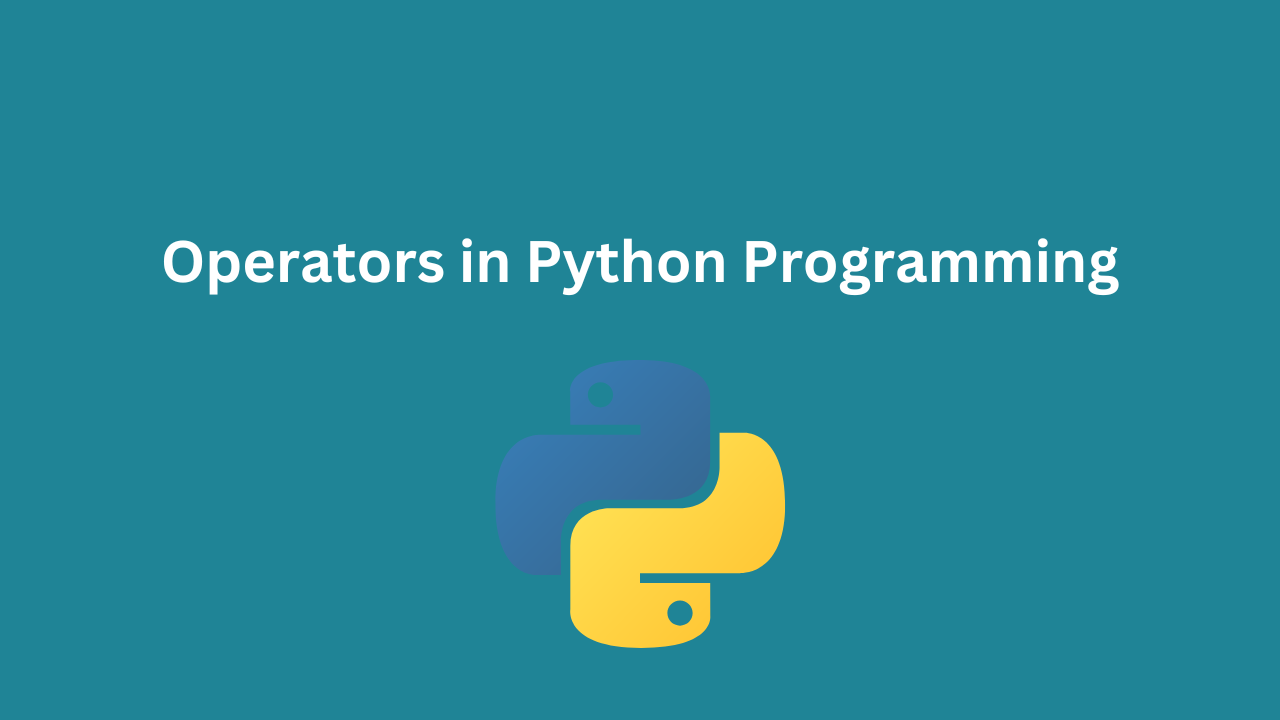
Introduction
Operators are an integral part of any programming language. These can be explained as special symbols or keywords taken in performance of operations on variables and values. Understanding and using operators effectively is the basics of writing efficient and effective Python code.
Arithmetic Operators
Addition (+)
This is an operator that adds these two operands. For example:
a = 5 b = 3 c = a + b print(c) # Output: 8
Subtraction (-)
The subtraction operator subtracts the second operand from the first. For example:
a = 5
b = 3
c = a - b
print(c) # Output: 2
Multiplication (*)
The multiplication operator multiplies two operands. For example:
a = 5 b = 3 c = a * b print(c) # Output: 15
Division (/)
The division operator divides the first operand by the second. For example:
a = 5 b = 3 c = a / b print(c) # Output: 1.6666666666666667
Modulus (%)
The modulus operator returns the remainder of the division of the first operand by the second. For example:
a = 5 b = 3 c = a % b print(c) # Output: 2
Exponentiation (**)
The exponentialization operator raises the first operand to the power of the second. For example:
a = 5 b = 3 c = a ** b print(c) # Output: 125
Floor Division (//)
The floor division operator divides the first operand by the second and returns the largest integer less than or equal to the result. For example:
a = 5 b = 3 c = a // b print(c) # Output: 1
Assignment Operators
Assignment (=)
The assignment operator assigns a value to a variable. For example:
a = 5
Add AND (+=)
The add AND operator adds the right operand to the left operand and assigns the result to the left operand. For example:
a = 5 a += 3 print(a) # Output: 8
Subtract AND (-=)
The subtract AND operator subtracts the right operand from the left operand and assigns the result to the left operand. For example:
a = 5 a -= 3 print(a) # Output: 2
Multiply AND (*=)
The multiply AND operator multiplies the right operand by the left operand and assigns the result to the left operand. For example:
a = 5 a *= 3 print(a) # Output: 15
Divide AND (/=)
The divide AND operator divides the left operand by the right operand and assigns the result to the left operand. For example:
a = 5 a /= 3 print(a) # Output: 1.6666666666666667
Modulus AND (%=)
The modulus AND operator takes the modulus using two operands and assigns the result to the left operand. For example:
a = 5 a %= 3 print(a) # Output: 2
Exponentiation AND (**=)
The exponentiation AND operator raises the left operand to the power of the right operand and assigns the result to the left operand. For example:
a = 5 a **= 3 print(a) # Output: 125
Floor Division AND (//=)
The floor division AND operator divides the left operand by the right operand and assigns the result to the left operand. For example:
a = 5 a //= 3 print(a) # Output: 1
Comparison Operators
Equal (==)
The equal operator checks if the values of two operands are equal. For example:
a = 5 b = 5 print(a == b) # Output: True
Not Equal (!=)
The not equal operator checks if the values of two operands are not equal. For example:
a = 5 b = 3 print(a != b) # Output: True
Greater Than (>)
The greater than operator checks if the value of the left operand is greater than the value of the right operand. For example:
a = 5 b = 3 print(a > b) # Output: True
Less Than (<)
The less than operator checks if the value of the left operand is less than the value of the right operand. For example:
a = 5 b = 3 print(a < b) # Output: False
Greater Than or Equal To (>=)
The greater than or equal to operator checks if the value of the left operand is greater than or equal to the value of the right operand. For example:
a = 5 b = 3 print(a >= b) # Output: True
Less Than or Equal To (<=)
The less than or equal to operator checks if the value of the left operand is less than or equal to the value of the right operand. For example:
a = 5 b = 3 print(a <= b) # Output: False
Logical Operators
AND
The AND operator returns True if both operands are true. For example:
a = True b = False print(a and b) # Output: False
OR
The OR operator returns True if at least one of the operands is true. For example:
a = True b = False print(a or b) # Output: True
NOT
The NOT operator returns True if the operand is false. For example:
a = True print(not a) # Output: False
Read also: C Language Online Compiler
Bitwise Operators
AND (&)
The AND operator performs a bitwise AND operation. For example:
a = 5 # 0101 b = 3 # 0011 print(a & b) # Output: 1 (0001)
OR (|)
The OR operator performs a bitwise OR operation. For example:
a = 5 # 0101 b = 3 # 0011 print(a | b) # Output: 7 (0111)
XOR (^)
The XOR operator performs a bitwise XOR operation. For example:
a = 5 # 0101 b = 3 # 0011 print(a ^ b) # Output: 6 (0110)
NOT (~)
The NOT operator performs a bitwise NOT operation. For example:
a = 5 # 0101 print(~a) # Output: -6 (inverts all bits)
Left Shift (<<)
The left shift operator shifts the bits of the first operand to the left by the number of positions specified by the second operand. For example:
a = 5 # 0101 print(a << 1) # Output: 10 (1010)
Right Shift (>>)
The right shift operator shifts the bits of the first operand to the right by the number of positions specified by the second operand. For example:
a = 5 # 0101 print(a >> 1) # Output: 2 (0010)
Membership Operators
in
The in operator checks if a value is present in a sequence. For example:
a = [1, 2, 3, 4, 5] print(3 in a) # Output: True
not in
The not in operator checks if a value is not present in a sequence. For example:
a = [1, 2, 3, 4, 5] print(6 not in a) # Output: True
Identity Operators
is
The is operator checks if two variables point to the same object. For example:
a = [1, 2, 3] b = a print(a is b) # Output: True
is not
The is not operator checks if two variables do not point to the same object. For example:
a = [1, 2, 3] b = [1, 2, 3] print(a is not b) # Output: True
Operator Precedence
Understanding Operator Precedence
Operator precedence determines the order in which operators are evaluated. For example, multiplication is evaluated before addition.
Assess your python scripts and execute the compiled versions with web-based platforms like Python Online Compiler.
Examples of Operator Precedence
a = 5 + 3 * 2 print(a) # Output: 11 (multiplication is done first)
Special Operators
Ternary Operator
The ternary operator is a shortcut for the if-else statement. For example:
a = 5 b = 3 min_val = a if a < b else b print(min_val) # Output: 3
Walrus Operator
The walrus operator allows assignment within an expression. For example:
a = 5 print(b := a + 2) # Output: 7
Overloading Operators
What is Operator Overloading?
Operator overloading allows custom behavior for operators when applied to user-defined types.
Overloading Arithmetic Operators
class Point: def init(self, x, y): self.x = x self.y = y def add(self, other): return Point(self.x + other.x, self.y + other.y)
Overloading Comparison Operators
class Point: def init(self, x, y): self.x = x self.y = y def eq(self, other): return self.x == other.x and self.y == other.y
Applications of Operators
Using Operators in Conditional Statements
a = 5 if a > 3: print("a is greater than 3")
Operators in Loops
for i in range(5): print(i)
Operators in List Comprehensions
a = [1, 2, 3, 4, 5] squares = [x**2 for x in a] print(squares) # Output: [1, 4, 9, 16, 25]
Operators in Functions
def add(a, b): return a + b print(add(5, 3)) # Output: 8
Best Practice when Using Operators
Readability in Writing operators
Use parentheses to explicitly set precedence.
Avoid overloading operators unnecessarily.
Common pitfalls when using operators
Be careful with division involving floating-point numbers.
Remember that == is not the same thing as is.
Testing and Debugging your usage of operators
Write unit tests which check operator behavior.
Use print statements to help debug complicated expressions.
Conclusion
Mastering operators in the language is quite indispensable for any efficient and effective Python programming. Operators are used in every aspect of coding—from simple arithmetic to complex logic. Learning how to use them, their order of precedence, and the best practices will enable a user to write clean, readable code.
Frequently Asked Questions
What are the most frequently used operators in Python?
The most common operators are arithmetic operators like +, -, *, /, comparison operators such as ==, !=, >, <.
How are logical operators used in Python?
The logical operators and, or, and are not used to connect conditional statements.
What is the difference between == and is in Python?
== checks for equality of values and is checks for identity .
How do you overload operators in Python?
Overloading can be done by defining some special methods in the class. These are add for the + operator and eq for the == operator.
Could you explain operator precedence in Python?
The order of these operations is defined by operator precedence. In the expression, 5 + 3 * 2 the multiplication will be executed before the addition. This will return 11.
Write a comment ...